Directives
Directives are special attributes with the v- prefix. Vue provides a number of built-in directives, including v-html and v-bind which we have introduced above.
Directive attribute values are expected to be single JavaScript expressions (with the exception of v-for, v-on and v-slot, which will be discussed in their respective sections later). A directive's job is to reactively apply updates to the DOM when the value of its expression changes. Take v-if as an example:
<p v-if="seen">Now you see me</p>
Here, the v-if directive would remove / insert the <p> element based on the truthiness of the value of the expression seen.
Arguments
Some directives can take an "argument", denoted by a colon after the directive name. For example, the v-bind directive is used to reactively update an HTML attribute:
<a v-bind:href="url"> ... </a>
<!-- shorthand -->
<a :href="url"> ... </a>
Here, href is the argument, which tells the v-bind directive to bind the element's href attribute to the value of the expression url. In the shorthand, everything before the argument (i.e., v-bind:) is condensed into a single character, :.
Another example is the v-on directive, which listens to DOM events:
<a v-on:click="doSomething"> ... </a>
<!-- shorthand -->
<a @click="doSomething"> ... </a>
Here, the argument is the event name to listen to: click. v-on has a corresponding shorthand, namely the @ character. We will talk about event handling in more detail too.
Dynamic Arguments
It is also possible to use a JavaScript expression in a directive argument by wrapping it with square brackets:
<!--
Note that there are some constraints to the argument expression,
as explained in the "Dynamic Argument Value Constraints" and "Dynamic Argument Syntax Constraints" sections below.
-->
<a v-bind:[attributeName]="url"> ... </a>
<!-- shorthand -->
<a :[attributeName]="url"> ... </a>
Here, attributeName will be dynamically evaluated as a JavaScript expression, and its evaluated value will be used as the final value for the argument. For example, if your component instance has a data property, attributeName, whose value is "href", then this binding will be equivalent to v-bind:href.
Similarly, you can use dynamic arguments to bind a handler to a dynamic event name:
<a v-on:[eventName]="doSomething"> ... </a>
<!-- shorthand -->
<a @[eventName]="doSomething">
In this example, when eventName's value is "focus", v-on:[eventName] will be equivalent to v-on:focus.
Dynamic Argument Value Constraints
Dynamic arguments are expected to evaluate to a string, with the exception of null. The special value null can be used to explicitly remove the binding. Any other non-string value will trigger a warning.
Dynamic Argument Syntax Constraints
Dynamic argument expressions have some syntax constraints because certain characters, such as spaces and quotes, are invalid inside HTML attribute names. For example, the following is invalid:
<!-- This will trigger a compiler warning. -->
<a :['foo' + bar]="value"> ... </a>
If you need to pass a complex dynamic argument, it's probably better to use a computed property, which we will cover shortly.
When using in-DOM templates (templates directly written in an HTML file), you should also avoid naming keys with uppercase characters, as browsers will coerce attribute names into lowercase:
<a :[someAttr]="value"> ... </a>
The above will be converted to :[someattr] in in-DOM templates. If your component has a someAttr property instead of someattr, your code won't work. Templates inside Single-File Components are not subject to this constraint.
Modifiers
Modifiers are special postfixes denoted by a dot, which indicate that a directive should be bound in some special way. For example, the .prevent modifier tells the v-on directive to call event.preventDefault() on the triggered event:
<form @submit.prevent="onSubmit">...</form>
You'll see other examples of modifiers later, for v-on and for v-model, when we explore those features.
And finally, here's the full directive syntax visualized:
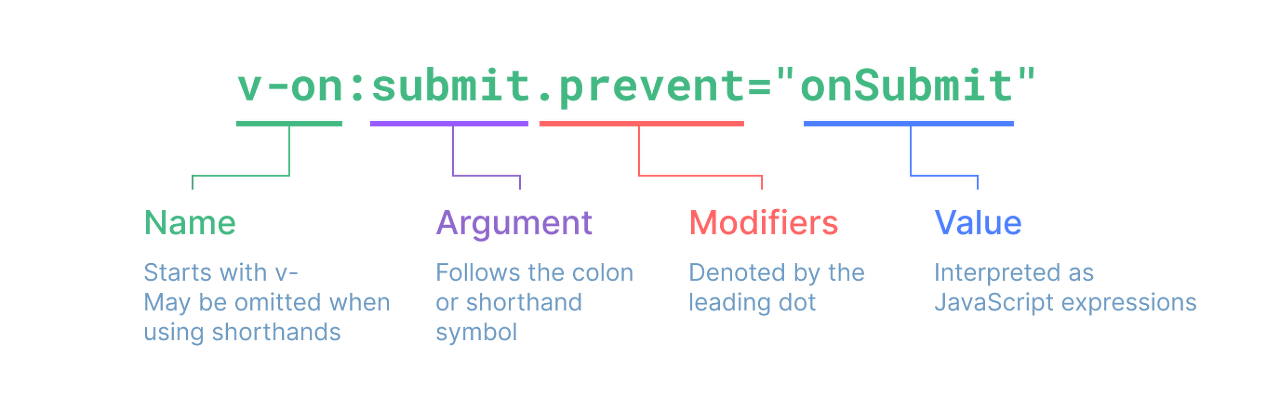
'Web > front' 카테고리의 다른 글
[mozilla WEB API Element] keydown_event (0) | 2023.08.24 |
---|---|
[Scope & Closure] (0) | 2023.04.20 |
[Vue.js] Template Syntax (1) (0) | 2023.04.12 |
[Vue.js] What is Vue? (0) | 2023.04.11 |
[Thymeleaf + Spring] 18 Appendix A: Expression Basic Objects (0) | 2023.02.20 |